mirror of
https://github.com/metafy-social/python-scripts.git
synced 2025-04-06 21:05:54 +00:00
commit
2db16fc2ea
12
scripts/RSA_algo_EtoE_Encryption/README.md
Normal file
12
scripts/RSA_algo_EtoE_Encryption/README.md
Normal file
@ -0,0 +1,12 @@
|
||||
# RSA ENCRYPTION ALGO IMPLEMENTATION IN PYTHON
|
||||
|
||||
- I've imported the rsa package.
|
||||
- It generates the public and private key.
|
||||
- It then stores the keys in keys/privkey.pem and keys/publickey.pem.
|
||||
- The keys are then loaded to the program as publickey and privatekey.
|
||||
- The inputed text or message is encrypted with the public key.
|
||||
- Which then is put in the variable ciphertext.
|
||||
- The private key is used to decrypt the message.
|
||||
- Here we also verify the signature of the message and the keys, whether they are signified or not.
|
||||
|
||||
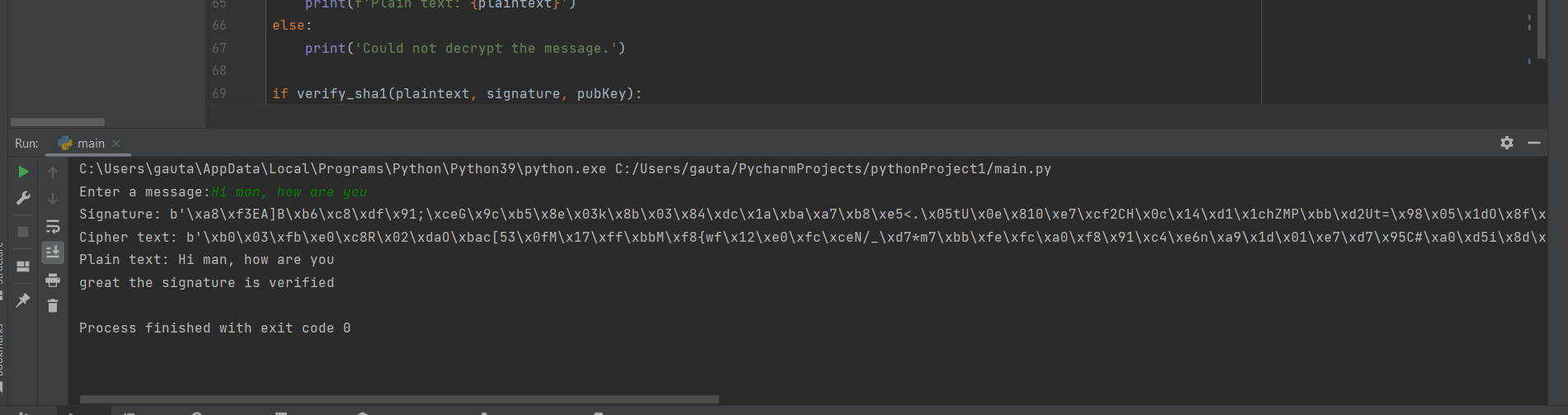
|
1
scripts/RSA_algo_EtoE_Encryption/keys/privkey.pem
Normal file
1
scripts/RSA_algo_EtoE_Encryption/keys/privkey.pem
Normal file
@ -0,0 +1 @@
|
||||
|
1
scripts/RSA_algo_EtoE_Encryption/keys/pubkey.pem
Normal file
1
scripts/RSA_algo_EtoE_Encryption/keys/pubkey.pem
Normal file
@ -0,0 +1 @@
|
||||
|
73
scripts/RSA_algo_EtoE_Encryption/main.py
Normal file
73
scripts/RSA_algo_EtoE_Encryption/main.py
Normal file
@ -0,0 +1,73 @@
|
||||
import rsa
|
||||
|
||||
##this function generates the keys
|
||||
def generate_keys():
|
||||
(pubKey, privKey) = rsa.newkeys(1024)
|
||||
with open('keys/pubkey.pem', 'wb') as f:
|
||||
f.write(pubKey.save_pkcs1('PEM'))
|
||||
|
||||
with open('keys/privkey.pem', 'wb') as f:
|
||||
f.write(privKey.save_pkcs1('PEM'))
|
||||
|
||||
##Loads the keys from the .pem files to pubkey and privkey variables
|
||||
def load_keys():
|
||||
with open('keys/pubkey.pem', 'rb') as f:
|
||||
pubKey = rsa.PublicKey.load_pkcs1(f.read())
|
||||
|
||||
with open('keys/privkey.pem', 'rb') as f:
|
||||
privKey = rsa.PrivateKey.load_pkcs1(f.read())
|
||||
|
||||
return pubKey, privKey
|
||||
|
||||
##encrypts the messages using the public key
|
||||
def encrypt(msg, key):
|
||||
return rsa.encrypt(msg.encode('ascii'), key)
|
||||
|
||||
##decrypts the encrypted message or ciphertext using the privatekey
|
||||
def decrypt(ciphertext, key):
|
||||
try:
|
||||
return rsa.decrypt(ciphertext, key).decode('ascii')
|
||||
except:
|
||||
return False
|
||||
|
||||
##the sha is generated for the message and key
|
||||
def sign_sha1(msg, key):
|
||||
return rsa.sign(msg.encode('ascii'), key, 'SHA-1')
|
||||
|
||||
##this function verifies the sha
|
||||
def verify_sha1(msg, signature, key):
|
||||
try:
|
||||
return rsa.verify(msg.encode('ascii'), signature, key) == 'SHA-1'
|
||||
except:
|
||||
return False
|
||||
|
||||
##generates keys
|
||||
generate_keys()
|
||||
|
||||
##loads keys
|
||||
pubKey, privKey = load_keys()
|
||||
|
||||
##Takes in the message and encrypts it
|
||||
message = input('Enter a message:')
|
||||
ciphertext = encrypt(message, pubKey)
|
||||
|
||||
signature = sign_sha1(message, privKey)
|
||||
|
||||
##It then decrypts the message.
|
||||
plaintext = decrypt(ciphertext, privKey)
|
||||
|
||||
##the sgnature of the message is displayed
|
||||
print(f'Signature: {signature}')
|
||||
##the cipher of the message is displayed
|
||||
print(f'Cipher text: {ciphertext}')
|
||||
|
||||
##the decrypted message is displayed
|
||||
if plaintext:
|
||||
print(f'Plain text: {plaintext}')
|
||||
else:
|
||||
print('Could not decrypt the message.')
|
||||
|
||||
if verify_sha1(plaintext, signature, pubKey):
|
||||
print('great the signature is verified')
|
||||
else:
|
||||
print('Could not verify the message signature.')
|
Loading…
x
Reference in New Issue
Block a user